Understanding Transformers in Laravel: A Complete Guide with Examples
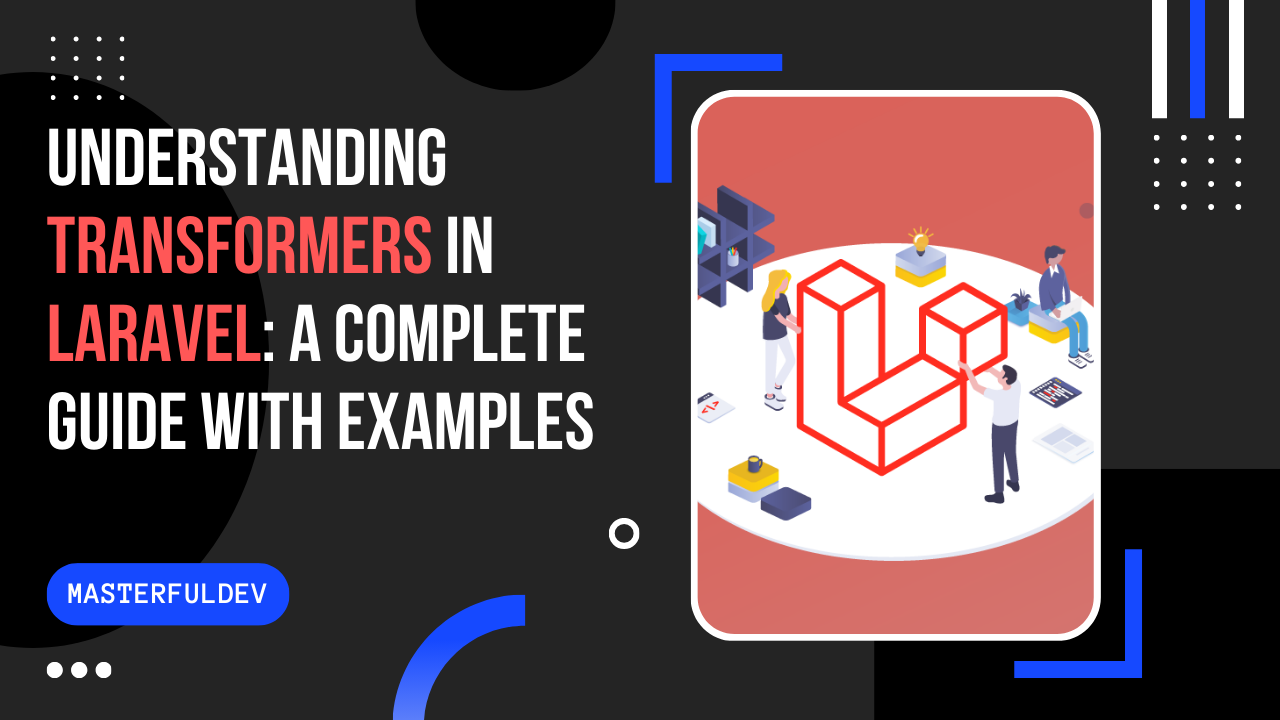
Summary
- Introduction
- What Are Transformers?
- Why Should You Use Transformers?
- Getting Started with Transformers in Laravel
- Step 1: Install Laravel Fractal
- Step 2: Create a Transformer
- Step 3: Use the Transformer in a Controller
- Step 4: Handling Collections
- Step 5: Including Relationships
- Step 6: Customizing Includes Based on Context
- Conclusion
- References
Introduction
When building APIs in Laravel, ensuring that your data is consistently formatted and secure is a top priority. This is where Transformers come into play. Transformers allow you to format your data into a consistent structure that can be easily consumed by your frontend or third-party services.
In this article, we’ll dive deep into what transformers are, why they’re essential, and how you can implement them in Laravel using Fractal.
What Are Transformers?
In Laravel, transformers are classes responsible for transforming your model data into a specific format before it’s sent out in API responses. They help to:
- Maintain consistency in API responses.
- Separate data transformation logic from your business logic.
- Ensure security by controlling what data gets exposed.
- Facilitate easier maintenance and scaling of your application.
Why Should You Use Transformers?
Transformers are particularly useful for:
- Consistency: All API responses follow a consistent structure, making it easier for frontend developers or external consumers to work with your API.
- Security: By selectively exposing only the necessary fields, you reduce the risk of sensitive data being unintentionally exposed.
- Flexibility: Need to add or remove fields, or change the format of the data? Transformers make this process straightforward without altering your core logic.
Getting Started with Transformers in Laravel
We’ll use Laravel Fractal to implement transformers. Fractal is a powerful package for transforming data, and Laravel Fractal provides an easy way to integrate it with your Laravel application.
Step 1: Install Laravel Fractal
First, install the Laravel Fractal package using Composer:
1
composer require spatie/laravel-fractal
Once installed, you can optionally publish the configuration file:
1
php artisan vendor:publish --provider="Spatie\Fractal\FractalServiceProvider"
Step 2: Create a Transformer
Let’s create a transformer for a User model. Transformers typically extend League\Fractal\TransformerAbstract and define a transform method to specify how the model’s attributes should be transformed.
Here’s an example:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
<?php
namespace App\Transformers;
use League\Fractal\TransformerAbstract;
use App\Models\User;
class UserTransformer extends TransformerAbstract
{
public function transform(User $user)
{
return [
'id' => $user->id,
'name' => $user->name,
'email' => $user->email,
'registered_at' => $user->created_at->format('Y-m-d H:i:s'),
];
}
}
In this example, we’re transforming the User model to expose only the id, name, email, and created_at fields (formatted as registered_at).
Step 3: Use the Transformer in a Controller
Now that we have our transformer, we can use it in our controller to transform the data before returning it in the API response.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
<?php
namespace App\Http\Controllers;
use App\Models\User;
use App\Transformers\UserTransformer;
use Spatie\Fractal\Fractal;
use Illuminate\Http\Request;
class UserController extends Controller
{
public function show($id)
{
$user = User::findOrFail($id);
return fractal($user, new UserTransformer())->toArray();
}
}
In this example, the show method fetches a user by ID and transforms the data using the UserTransformer before returning it in the API response.
Step 4: Handling Collections
If you need to transform a collection of users, you can use the collection method provided by Fractal.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
<?php
namespace App\Http\Controllers;
use App\Models\User;
use App\Transformers\UserTransformer;
use Spatie\Fractal\Fractal;
class UserController extends Controller
{
public function index()
{
$users = User::all();
return fractal()
->collection($users)
->transformWith(new UserTransformer())
->toArray();
}
}
This will transform all users in the collection and return them in the desired format.
Step 5: Including Relationships
One of the powerful features of Fractal is the ability to include related data (e.g., posts, comments) in your API responses. You can define these includes in your transformer and conditionally include them in the response.
For example, if you want to include a user’s posts in the response:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
<?php
namespace App\Transformers;
use League\Fractal\TransformerAbstract;
use App\Models\User;
use App\Transformers\PostTransformer;
class UserTransformer extends TransformerAbstract
{
protected $defaultIncludes = ['posts'];
public function transform(User $user)
{
return [
'id' => $user->id,
'name' => $user->name,
'email' => $user->email,
];
}
public function includePosts(User $user)
{
$posts = $user->posts;
return $this->collection($posts, new PostTransformer());
}
}
Then, in your controller, you can specify whether or not to include the posts:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
<?php
namespace App\Http\Controllers;
use App\Models\User;
use App\Transformers\UserTransformer;
use Spatie\Fractal\Fractal;
use Illuminate\Http\Request;
class UserController extends Controller
{
public function show($id)
{
$user = User::findOrFail($id);
return fractal()
->item($user)
->transformWith(new UserTransformer())
->parseIncludes(['posts'])
->toArray();
}
}
Step 6: Customizing Includes Based on Context
You can customize what data gets included or excluded based on different contexts, such as user roles, API versions, or request parameters. This provides flexibility in tailoring your API responses to specific use cases.
Conclusion
Transformers are a powerful tool in Laravel that help you manage data transformation in a clean, consistent, and secure way. By using transformers, you separate data formatting logic from your core business logic, making your code easier to maintain and scale.
Laravel Fractal simplifies the process of implementing transformers and handling complex data structures, making it an essential part of building robust APIs.
Whether you’re just starting with API development or looking to improve your existing application, integrating transformers into your workflow can make a significant difference.
References