Enhancing Microservice Communication: Real-Time Order Processing with RabbitMQ and Kafka
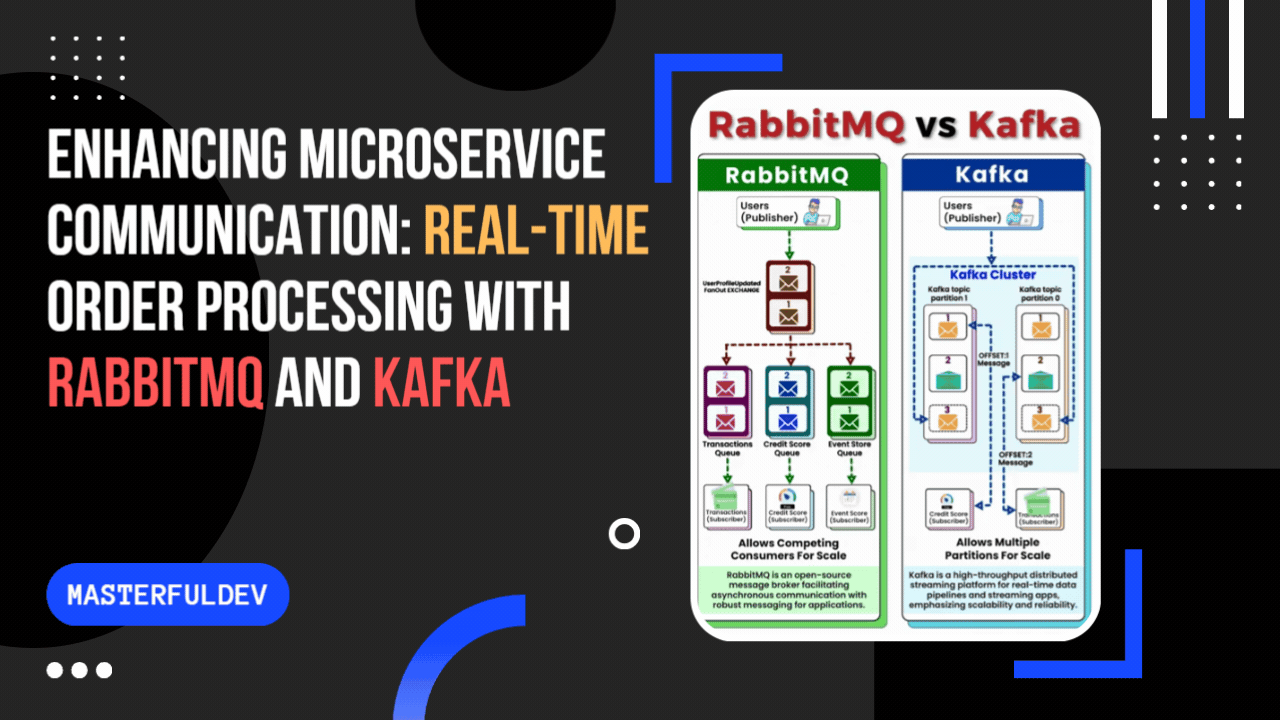
Using RabbitMQ or Kafka for communication between microservices can be advantageous in scenarios where high throughput, fault tolerance, and real-time data processing are required. Here’s a concrete example:
Real-Time Order Processing in an E-commerce Platform
Scenario: An e-commerce platform handles high volumes of user transactions, including placing orders, processing payments, updating inventory, and sending notifications.
REST API Approach:
Using REST APIs, each microservice (Order, Payment, Inventory, Notification) communicates directly with each other. When a user places an order:
- The Order service calls the Payment service via a REST API to process the payment.
- Once the payment is confirmed, the Payment service calls the Inventory service to update stock levels.
- The Inventory service then calls the Notification service to send a confirmation email to the user.
Challenges with REST API:
- High Latency: Each service-to-service call adds latency, making the overall process slower.
- Tight Coupling: Services are tightly coupled, and a failure in one service (e.g., Payment) can cascade to others, causing the entire order process to fail.
- Scalability Issues: Handling high volumes of orders can lead to REST API endpoints becoming bottlenecks, impacting performance.
RabbitMQ or Kafka Approach:
Using a message broker like RabbitMQ or Kafka can address these issues. Here’s how the workflow changes:
- Order Service: When a user places an order, the Order service publishes an OrderPlaced event to a message queue (RabbitMQ) or topic (Kafka).
- Payment Service: The Payment service subscribes to OrderPlaced events. Upon receiving an event, it processes the payment and publishes a PaymentProcessed event.
- Inventory Service: The Inventory service subscribes to PaymentProcessed events. Upon receiving an event, it updates the inventory and publishes an InventoryUpdated event.
- Notification Service: The Notification service subscribes to InventoryUpdated events. Upon receiving an event, it sends a confirmation email to the user.
Advantages of Using RabbitMQ or Kafka:
- Decoupling: Services are loosely coupled. They only need to know about the messages they publish or consume, not about other services.
- Asynchronous Processing: Services process messages asynchronously, improving response times and overall system performance.
- Scalability: Message brokers handle high volumes of messages efficiently, enabling better scalability.
- Fault Tolerance: Message brokers provide mechanisms for message persistence and retries, improving fault tolerance. If a service fails, messages can be retried or processed later.
Example of Event Flow:
- OrderPlaced Event:
1 2 3 4 5 6 7 8 9
{ "event": "OrderPlaced", "orderId": "12345", "userId": "user1", "items": [ {"productId": "abc", "quantity": 2}, {"productId": "xyz", "quantity": 1} ] }
- PaymentProcessed Event:
1 2 3 4 5 6
{ "event": "PaymentProcessed", "orderId": "12345", "paymentStatus": "confirmed", "paymentId": "pay789" }
- InventoryUpdated Event:
1 2 3 4 5
{ "event": "InventoryUpdated", "orderId": "12345", "status": "items reserved" }
Using RabbitMQ or Kafka in such a scenario leads to a more resilient, scalable, and performant microservice architecture compared to relying solely on REST APIs for inter-service communication.