Mastering Laravel's Transform Helper: A Comprehensive Guide
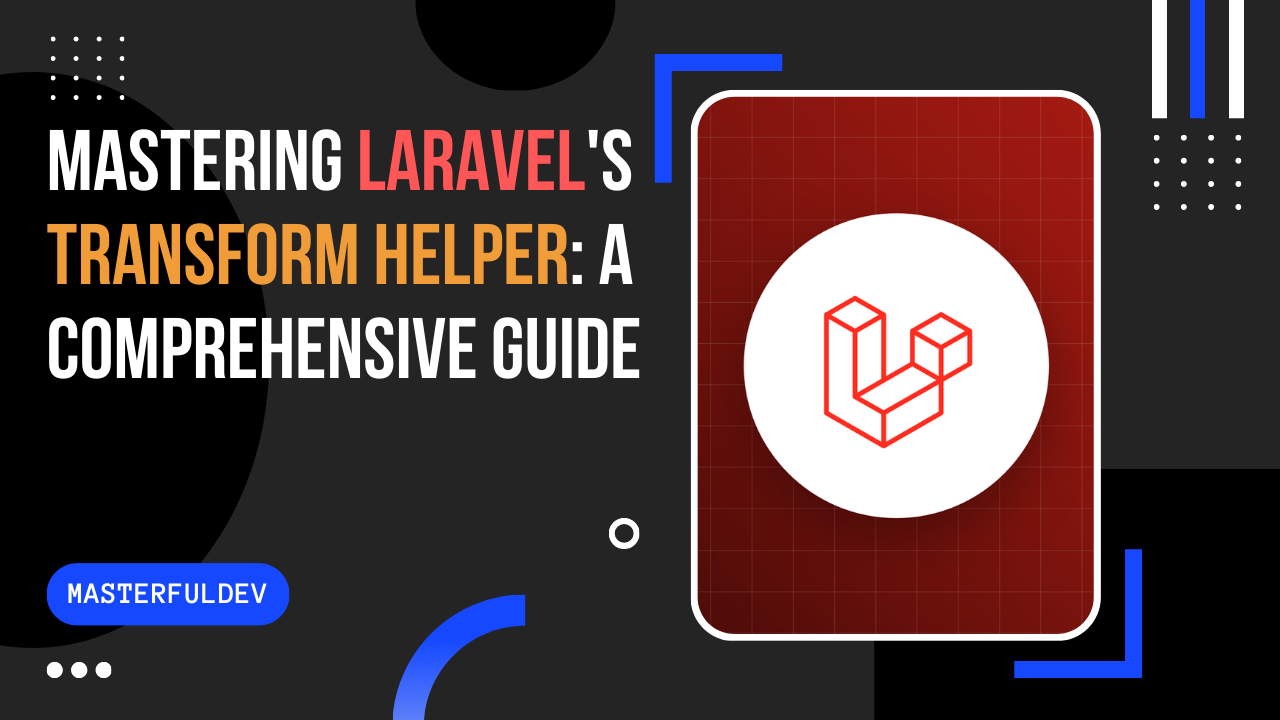
Summary
Laravel’s transform helper is a powerful tool for modifying data in your application. Introduced in Laravel 5.1, it has become an essential part of many developers’ toolkits. In this article, we’ll dive deep into the transform helper, exploring its functionality, use cases, and best practices.
What is the Transform Helper?
The transform helper is a function that allows you to apply a callback to each item in a collection or array. It’s particularly useful for formatting data, preparing it for API responses, or modifying it before saving to a database.
Transform Helper Syntax and Parameters
The full syntax of the transform helper includes optional parameters that provide additional functionality:
1
transform($value, callable $callback = null, $default = null)
Parameters:
- $value (mixed):
- The value to be transformed. This can be an array, a collection, or any other type of value.
- $callback (callable/null):
- The callback function to apply to each element.
- If null, the transform helper acts as a getter and returns the original value.
- $default (mixed):
- The default value to return if $value is null.
Basic Usage
The basic syntax of the transform helper is as follows:
1
2
3
4
$result = transform($data, function ($item) {
// Modify $item
return $modifiedItem;
});
Here, $data
can be an array or a collection, and the callback function is applied to each item.
Detailed Examples
Transforming User Data
Let’s consider a scenario where we need to format user data for an API response:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
use Illuminate\Support\Carbon;
$users = [
[
'id' => 1,
'name' => 'John Doe',
'email' => 'john@example.com',
'created_at' => '2023-09-15 10:30:00',
'is_admin' => true,
],
[
'id' => 2,
'name' => 'Jane Smith',
'email' => 'jane@example.com',
'created_at' => '2023-10-20 14:45:00',
'is_admin' => false,
],
];
$transformedUsers = transform($users, function ($user) {
return [
'id' => $user['id'],
'full_name' => $user['name'],
'email_address' => $user['email'],
'joined_date' => Carbon::parse($user['created_at'])->format('F j, Y'),
'role' => $user['is_admin'] ? 'Administrator' : 'User',
];
});
print_r($transformedUsers);
In this example, we:
- Rename some keys for clarity
- Format the
created_at
date - Convert the boolean
is_admin
to a more descriptive role
Working with Different Value Types
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
// Array
$result = transform([1, 2, 3], function ($item) {
return $item * 2;
});
// Result: [2, 4, 6]
// Collection
$collection = collect(['a', 'b', 'c']);
$result = transform($collection, function ($item) {
return strtoupper($item);
});
// Result: ['A', 'B', 'C']
// Single value
$result = transform('hello', function ($item) {
return strrev($item);
});
// Result: 'olleh'
// Null value
$result = transform(null, function ($item) {
return $item * 2;
});
// Result: null
Using the Callback Parameter
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
// Basic callback
$result = transform([1, 2, 3], function ($item) {
return $item * 2;
});
// Result: [2, 4, 6]
// Callback with key
$result = transform(['a' => 1, 'b' => 2], function ($value, $key) {
return "$key: $value";
});
// Result: ['a: 1', 'b: 2']
// Null callback (acts as a getter)
$result = transform([1, 2, 3], null);
// Result: [1, 2, 3]
Using the Default Parameter
1
2
3
4
5
6
7
8
9
10
11
// With null value and default
$result = transform(null, function ($item) {
return $item * 2;
}, 'No data');
// Result: 'No data'
// With non-null value and default (default is ignored)
$result = transform(5, function ($item) {
return $item * 2;
}, 'No data');
// Result: 10
Advanced Usage
Transforming Eloquent Collections
1
2
3
4
5
6
7
8
9
10
11
12
13
14
use App\Models\Post;
$posts = Post::all();
$transformedPosts = transform($posts, function ($post) {
return [
'id' => $post->id,
'title' => $post->title,
'excerpt' => Str::limit($post->content, 100),
'author' => $post->author->name,
'published_at' => $post->published_at->diffForHumans(),
'comments_count' => $post->comments->count(),
];
});
Conditional Transformation
1
2
3
4
5
6
7
8
9
10
11
12
13
$users = [
['name' => 'John', 'age' => 30],
['name' => 'Jane', 'age' => 25],
];
$result = transform($users, function ($user) {
if ($user['age'] >= 30) {
$user['category'] = 'Senior';
} else {
$user['category'] = 'Junior';
}
return $user;
});
Nested Transformations
1
2
3
4
5
6
7
8
9
10
11
12
13
14
$data = [
'users' => [
['name' => 'John', 'posts' => [1, 2, 3]],
['name' => 'Jane', 'posts' => [4, 5]],
]
];
$result = transform($data, function ($data) {
$data['users'] = transform($data['users'], function ($user) {
$user['post_count'] = count($user['posts']);
return $user;
});
return $data;
});
Using with Eloquent Relationships
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
use App\Models\User;
$users = User::with('posts')->get();
$result = transform($users, function ($user) {
return [
'id' => $user->id,
'name' => $user->name,
'email' => $user->email,
'posts' => transform($user->posts, function ($post) {
return [
'id' => $post->id,
'title' => $post->title,
'excerpt' => Str::limit($post->content, 100),
];
}),
];
});
Null Safety
One of the advantages of the transform helper is its null safety. If you pass null to the transform helper, it will return null without applying the callback:
1
2
3
4
5
$result = transform(null, function ($value) {
return strtoupper($value);
});
// $result will be null
Transform vs. Map
While the transform helper is similar to the map()
method available on collections, there are some key differences:
transform()
can work with both arrays and collections, whilemap()
is a method on collections.transform()
is null-safe, as mentioned earlier.map()
always returns a new Collection instance, whiletransform()
returns an array when given an array input.
Best Practices and Tips
- Use transform for data preparation: It’s ideal for formatting data before sending it to views or API responses.
- Keep transformations pure: Avoid side effects in your transform callbacks.
- Consider creating dedicated Transformer classes for complex transformations.
- Use type-hinting in your callback functions for better code readability and IDE support:
1
2
3
$result = transform($users, function (array $user): array {
// Transform logic here
});
- Chaining: You can chain multiple transformations for more complex operations:
1
2
3
4
5
6
7
$result = transform($data, function ($item) {
return strtoupper($item);
});
$result = transform($result, function ($item) {
return "Prefix_$item";
});
- Performance: For large datasets, consider using Laravel’s collection methods like
map()
directly, as they may offer better performance for certain operations. - Reusability: For frequently used transformations, consider creating dedicated Transformer classes or helper functions.
Conclusion
The transform helper in Laravel is a versatile tool that can significantly clean up your code when working with data transformations. Whether you’re preparing data for an API, formatting it for display, or modifying it before storage, the transform helper provides a clean and efficient way to handle these tasks.
Its ability to work with various data types, including arrays, collections, and single values, makes it a flexible choice for many scenarios. The null safety feature and optional default parameter add an extra layer of robustness to your code.
By mastering the transform helper and understanding its parameters and advanced techniques, you can write more expressive, flexible, and maintainable Laravel applications. It’s particularly useful in API development, data formatting, and when working with Eloquent models and relationships.
Remember to consider the best practices mentioned, such as using type-hinting, keeping transformations pure, and creating reusable transformer classes for complex operations. These practices will help you leverage the full power of the transform helper while maintaining clean and efficient code.
As you continue to work with Laravel, you’ll likely find many situations where the transform helper can simplify your code and make your data manipulations more elegant. It’s a powerful tool in the Laravel developer’s toolkit, and mastering it will undoubtedly enhance your productivity and code quality.